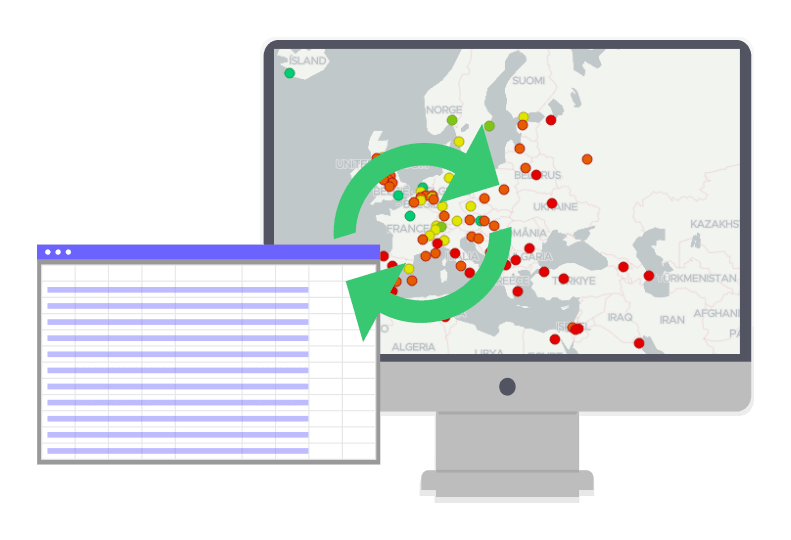
Bulk Convert List Of Addresses to Lat/Long with Geoapify Batch Geocoding
Batch Geocoding was designed to save you time and decrease your expenses when processing a large number of addresses. You can easily convert a list of addresses to latitude and longitude coordinates with one API call.
By getting our users geocoding requests as a batch, we can distribute our resources better to offer lower geocoding prices. As a result, processing one address with the batch geocoder costs 0.5 credits, while the regular geocoder requests cost 1 credit. So batch convert address to lat/long is 50% cheaper.
How Batch Geocoding service works
Batch geocoding is asynchronous. All you need to do is submit the addresses to convert to latitude and longitude coordinates and get results after some time.
Below is a diagram that illustrates how the bulk geocoding works:
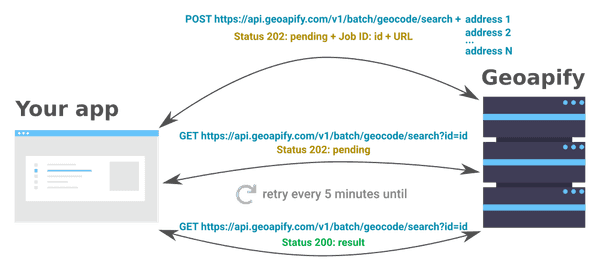
- To start batch geocoding process, you send a list of addresses to our servers using a simple HTTP POST request. You’ll see the job ID and URL to get the results when you get your response.
- Make an HTTP GET to this URL, get back the results. If the job has not finished processing, this call will return "pending" in the status field. Once the job has finished, the response will contain the results including the longitude and latitude of each address.
In general, the processing time depends on the number of addresses. You may check the status of your batch geocoding tasks by calling the results URL. But you don't have to wait until the batcher finishes its job. It’s possible and even recommended to create multiple jobs and then pick up the results later.
The results are available the next 24 hours after the job is completed.
How To Implement Batch Geocoding and Start Converting Addresses to Lat/Long in Bulk
We provide a simple RESTful API, so you can quickly get your addresses geocoded. You can process them with no coding, for example with Postman or CURL. Here is a Tutorial on how to to do that.
You can also do it programmatically. Here is a code example of how this could be implemented.
Start Batch Geocoder job
To use the Geoapify API you will need to generate an API key. Find out how to create an API Key on the Getting Started page.
Crease an HTTP POST request on the "https://api.geoapify.com/v1/batch/geocode/search
" and send the addresses to geocode as the request body as JSON array:
const data = [
"668 Cedar St, San Carlos, CA 94070, United States of America",
"545 Southwest Taylor Street, Portland, OR 97204, United States of America",
"1415 Southwest Park Avenue, Portland, OR 97201, United States of America"
];
const url = `https://api.geoapify.com/v1/batch/geocode/search?apiKey=YOUR_API_KEY`;
fetch(url, {
method: 'post',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(getBodyAndStatus)
.then((result) => {
if (result.status !== 202) {
return Promise.reject(result)
} else {
console.log("Job ID: " + result.body.id);
console.log("Job URL: " + result.body.url);
}
})
.catch(err => console.log(err));
Get results - addresses, their latitude and longitude, address components
This job is in progress now. To get results, use the URL you received in the response. It may take several attempts to get your result:
function getBodyAndStatus(response) {
return response.json().then(responceBody => {
return {
status: response.status,
body: responceBody
}
});
}
function getAsyncResult(url, timeout /*timeout between attempts*/, maxAttempt /*maximal amount of attempts*/) {
return new Promise((resolve, reject) => {
setTimeout(() => {
repeatUntilSuccess(resolve, reject, 0)
}, timeout);
});
function repeatUntilSuccess(resolve, reject, attempt) {
console.log("Attempt: " + attempt);
fetch(url)
.then(getBodyAndStatus)
.then(result => {
if (result.status === 200) {
resolve(result.body);
} else if (attempt >= maxAttempt) {
reject("Max amount of attempt achived");
} else if (result.status === 202) {
// Check again after timeout
setTimeout(() => {
repeatUntilSuccess(resolve, reject, attempt + 1)
}, timeout);
} else {
// Something went wrong
reject(result.body)
}
})
.catch(err => reject(err));
};
}
So now you can call the API and get results this way:
fetch(url, {
method: 'post',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(getBodyAndStatus)
.then((result) => {
if (result.status !== 202) {
return Promise.reject(result)
} else {
return getAsyncResult(`${url}&id=${result.body.id}`, 60 * 1000 /* 60 seconds */, 100).then(queryResult => {
console.log(queryResult);
return queryResult;
});
}
})
.catch(err => console.log(err));
You can run and try the code sample with JSFiddle.
Example of a result object
- {} 20 keys▶
- {} 2 keys▶
- "668 Cedar St, San Carlos, CA 94070, United States of America"
- {} 7 keys▶
- "668"
- "cedar st"
- "94070"
- "san carlos"
- "ca"
- "united states of america"
- "building"
- {} 4 keys▶
- "openstreetmap"
- "© OpenStreetMap contributors"
- "Open Database License"
- "https://www.openstreetmap.org/copyright"
- "668"
- "Cedar Street"
- "Devonshire"
- "San Carlos"
- "San Mateo County"
- "California"
- "94070"
- "United States of America"
- "us"
- -122.26380104761905
- 37.502683380952384
- "668 Cedar Street, San Carlos, CA 94070, United States of America"
- "668 Cedar Street"
- "San Carlos, CA 94070, United States of America"
- "CA"
- "building"
- {} 6 keys▶
- 1.021
- 4.377368426402045
- 1
- 1
- 1
- "full_match"
- "51290bca1de2905ec05973b7d4ed57c04240f00102f901f48dac0f00000000c00203"
Batch Geocoding parameters and features
Just like normal geocoding, batch geocoding accepts input parameters that serve for filtering or adjusting the output:
- type - location type, for example city, postcode, street, etc.;
- filter - filtering locations by geographical ares, for example, bounding box or country;
- bias - preferring locations by proximity;
- lang - to get localized version of addresses.
In addition, you can get results in JSON or CSV formats. Just add the format=csv to the resulting URL to get the results in a different form.
All details can be found on our documentation page.
Batch Geocoding tutorials
Check out our tutorials to make it easy to get started and get the results you want with Batch Geocoding:
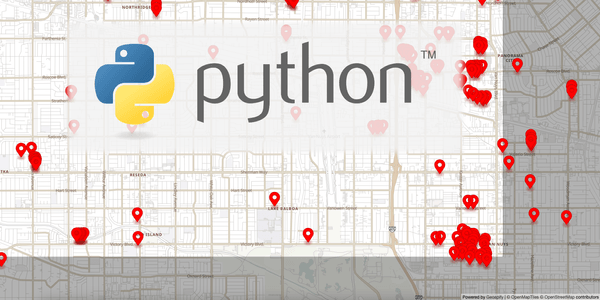
Geocoding in Python
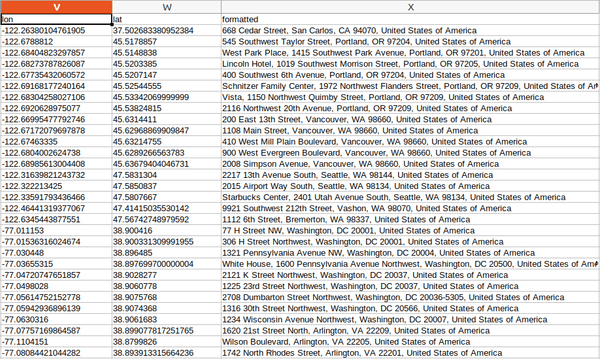
No-code Tutorial: Batch Geocode to CSV/JSON
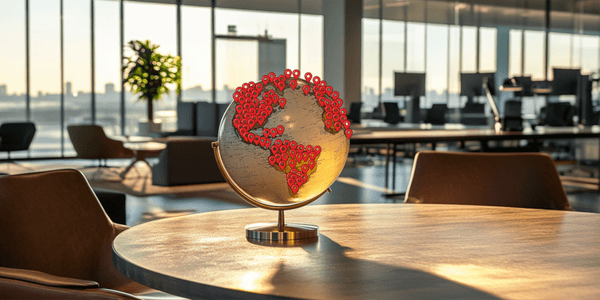
How To Geocode Millions Of Records Efficiently
Still not sure how to start? If you're facing any problems or have questions, we're here to help! Contact us and we will be happy to assist.
How much will batch geocoding cost you
The cost of batch geocoding depends on the number of addresses you’d like to convert to lat/long coordinates.
We give you a Free Plan for 6000 batch geocodes a day (or 3000 credits/day) with the option of five different paid plans to suit your needs.
For one-time jobs, we recommend subscribing to a plan that includes the required amount of credits and then canceling your subscription when you complete your work.
However, if you need specific, tailored solutions for you and your business, don’t hesitate to contact us for an offer.
FAQ
What is Batch Geocoding?
Batch geocoding or bulk geocoding is converting a list of addresses to well-formatted addresses with their geographical coordinates. In other words, batch up your list of addresses and look for latitude and longitude coordinates with one click or API call.
How to batch geocode addresses?
With Geoapify, you can batch geocode addresses using API or with no coding, for example, Postman. First, you need to call an HTTP POST request that contains the address to create a geocoding job. Then you get results by calling the provided URL. You can get results in JSON or CSV format.
How to geocode a huge batch of addresses?
The Geoapify Batch Geocoding accepts up to 1000 addresses at once. Programmatically split huge datasets of addresses into smaller batches and submit multiple batch jobs. Batch geocoding can take a long time as it involves a large number of data processing.